Change node tagName
Building up on the previous discovery about copying all node's attributes, I stumbled upon the need of having to rename the tag name of a DOM node. While this is not possible to do with stock DOM manipulation helpers, we can however follow this outline in order to achieve it:
- Create a empty node with the new tag name
- Copy over all the attributes from the old node (that's where we build upon the previous work)
- Move all the childs from the old node to the new one
- Replace the old node with the newly created one
The only downside to this is that we loose all the event listeners that were previously attached to the original DOM element. While this can most like be handled too, I won't cover that in this post.
That being said, let's look at the actual implementation of it.
function changeTagName(node, newTagName) {
// (1)
var renamed = document.createElement(newTagName);
// (2)
[...node.attributes].map(({ name, value }) => {
renamed.setAttribute(name, value);
});
// (3)
while (node.firstChild) {
renamed.appendChild(node.firstChild);
}
// (4)
return node.parentNode.replaceChild(renamed, node);
}
This is it and gets the job done in most cases, even if those are very rare.
I'd be curious to take a look at the code that implements the events listeners transfering, if possible at all.
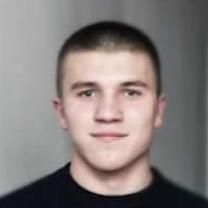
Andrei Glingeanu's notes and thoughts. You should follow him on Twitter, Instagram or contact via email. The stuff he loves to read can be found here on this site or on goodreads. Wanna vent or buy me a coffee?